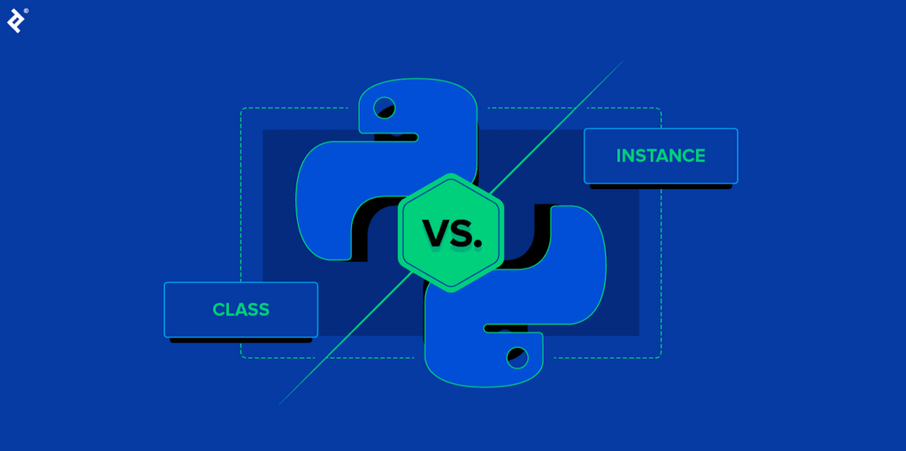
Another useful technique for the EDA of video data is to extract features from each frame and analyze them. Features are measurements or descriptors that capture some aspect of the image, such as color, texture, or shape. By analyzing these features, we can identify patterns and relationships in the data.
To extract features from each frame, we can use the OpenCV functions that compute various types of features, such as color histograms, texture descriptors, and shape measurements. Choosing the best feature extraction method depends on the characteristics of your data and the nature of the clustering task.
Let us see the color histogram feature extraction method.
Color histogram
A color histogram is a representation of the distribution of colors in an image. It shows the number of pixels that have different colors in each range of the color space. For example, a color histogram can show how many pixels are red, green, or blue in an image. Here is example code that extracts the color histogram from each frame and plots it:
import cv2
import matplotlib.pyplot as plt
video_path = “path/to/video.mp4”
cap = cv2.VideoCapture(video_path)
histograms = []
Here is a detailed explanation of each line in the code:
- The first line imports the cv2 library, which we will use to read and process video data.
- The second line imports the matplotlib library, which we will use to plot the histograms.
- The third line sets the path to the video file. Replace “path/to/video.mp4” with the actual path to your video file.
- The fourth line creates a VideoCapture object using the cv2.VideoCapture function. This object allows us to read frames from the video.
- The fifth line creates an empty list called histograms. We will store the histograms of each frame in this list.
Then, we add a while loop. The while loop reads frames from the video one by one until there are no more frames:
while True:
ret, frame = cap.read()
if not ret:
break
histogram = cv2.calcHist([frame], [0, 1, 2], \
None, [8, 8, 8], [0, 256, 0, 256, 0, 256])
histogram = cv2.normalize(histogram, None).flatten()
histograms.append(histogram)
cap.release()
Here is what each line inside the loop does:
- ret, frame = cap.read(): This line reads the next frame from the video using the cap.read() function. The ret variable is a Boolean value that indicates whether the frame was successfully read, and the frame variable is a NumPy array that contains the pixel values of the frame.
- if not ret: break: If ret is False, it means there are no more frames in the video, so we break out of the loop.
- histogram = cv2.calcHist([frame], [0, 1, 2], None, [8, 8, 8], [0, 256, 0, 256, 0, 256]): This line calculates the color histogram of the frame using the cv2.calcHist function. The first argument is the frame, the second argument specifies which channels to include in the histogram (in this case, all three RGB channels), the third argument is a mask (which we set to None), the fourth argument is the size of the histogram (8 bins per channel), and the fifth argument is the range of values to include in the histogram (0 to 256 for each channel).
- histogram = cv2.normalize(histogram, None).flatten(): This line normalizes the histogram using the cv2.normalize function and flattens it into a 1D array using the flatten method of the NumPy array. Normalizing the histogram ensures that it is scale-invariant and can be compared with histograms from other frames or videos.
- histograms.append(histogram): This line appends the histogram to the histograms list.
The final line releases the VideoCapture object using the cap.release() function. This frees up the resources used by the object and allows us to open another video file if we need to.