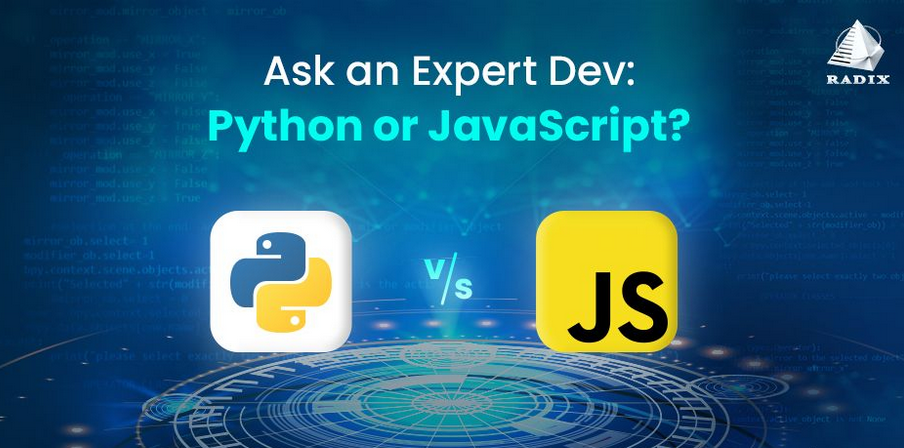
We will extract features based on the optical flow between consecutive frames. Optical flow captures the movement of objects in video. Libraries such as OpenCV provide functions to compute optical flow.
Let’s look at example code for optical flow features:
# Example of optical flow calculation
prev_frame = cv2.cvtColor(frame1, cv2.COLOR_BGR2GRAY)
next_frame = cv2.cvtColor(frame2, cv2.COLOR_BGR2GRAY)
flow = cv2.calcOpticalFlowFarneback(prev_frame, \
next_frame, None, 0.5, 3, 15, 3, 5, 1.2, 0)
Motion vectors
Motion vectors play a crucial role in understanding the dynamic aspects of video data. They represent the trajectory of key points or regions across frames, providing insights into the movement patterns within a video sequence. A common technique to calculate these motion vectors involves the use of Shi-Tomasi corner detection combined with Lucas-Kanade optical flow:
- Shi-Tomasi Corner Detection: In the following code, Shi-Tomasi corner detection is utilized to identify distinctive feature points in the initial frame (prev_frame). These feature points act as anchor points for tracking across subsequent frames.
- Lucas-Kanade Optical Flow: The Lucas-Kanade optical flow algorithm is then applied using cv2.calcOpticalFlowPyrLK. This algorithm estimates the motion vectors by calculating the flow of these feature points from the previous frame (prev_frame) to the current frame (next_frame).
We calculate motion vectors by tracking key points or regions across frames. These vectors represent the movement patterns in the video. Let’s see the example code for motion vectors:
# Example of feature tracking using Shi-Tomasi corner detection and Lucas-Kanade optical flow
corners = cv2.goodFeaturesToTrack(prev_frame, \
maxCorners=100, qualityLevel=0.01, minDistance=10)
next_corners, status, err = cv2.calcOpticalFlowPyrLK(\
prev_frame, next_frame, corners, None)
This code snippet demonstrates the initialization of feature points using Shi-Tomasi corner detection and subsequently calculating the optical flow to obtain the motion vectors. Understanding these concepts is fundamental for tasks such as object tracking and motion analysis in computer vision.
Deep learning features
Use features from pre-trained models other than VGG16, such as ResNet, Inception, or MobileNet. Experiment with models that are well-suited for image and video analysis. Implementation of these methods is beyond the scope of this book. You can find details in various deep learning documentation.
When working with pre-trained models such as ResNet, Inception, or MobileNet, you will find comprehensive documentation and examples from the respective deep learning frameworks. Here are some suggestions based on popular frameworks:
- TensorFlow documentation: TensorFlow provides detailed documentation and examples for using pre-trained models. You can explore TensorFlow Hub, which offers a repository of pre-trained models, including various architectures, such as ResNet, Inception, and MobileNet.
- Keras documentation: If you’re using Keras as part of TensorFlow, you can refer to the Keras Applications module. It includes pre-trained models such as ResNet50, InceptionV3, and MobileNet.
- PyTorch documentation: PyTorch provides documentation for using pre-trained models through the torchvision library. You can find ResNet, Inception, and MobileNet models, among others.
- Hugging Face Transformers library: For a broader range of pre-trained models, including those for natural language processing and computer vision, you can explore the Hugging Face Transformers library. It covers various architectures and allows easy integration into your projects.
- OpenCV deep neural networks (DNN) module: If you are working with OpenCV, the DNN module supports loading pre-trained models from frameworks such as TensorFlow, Caffe, and others. You can find examples and documentation on how to use these models.
By consulting these resources, you’ll find ample documentation, code examples, and guidelines for integrating pre-trained models into your image and video analysis tasks. Remember to check the documentation for the framework you are using in your project.